This example demonstrates the integration between ServiceNow and CoreView to fulfill an end-user request for creating a shared mailbox.
In this particular scenario, the user who requests the creation of the shared mailbox accesses a self-service catalog form in ServiceNow. They can enter the mailbox name and specify the users who should have access.
Part 1: creating a workflow in CoreView
The first step is to create a workflow in CoreView. If you don't know how to create a workflow, you can refer to the documentation or practice with this tutorial.
The workflow to create a Shared Mailbox involves several steps that can be omitted or customized according to your organizational business processes. In this example, steps for approvals have been omitted to simplify the workflow.
Elements of the workflow
In CoreView, create a workflow made by the following execution inputs and actions:
Consult the elements of the workflow
Inputs
Name | Type | Description |
---|---|---|
SharedMailboxName | String | The name of the Shared Mailbox to be created |
GroupMembers | String | The member or members to add for access to the Shared Mailbox |
ServiceNowID | String | The sys_id of the ServiceNow Request Item used to update the Request |
ServiceNowRequestID | String | The sys_id of the ServiceNow Request used to update the Request record |
Actions
Name | Description |
---|---|
CreateSharedMailbox | Creates the shared mailbox according to the name passed from ServiceNow |
AddMailboxPermissions | Adds the member or members passed in from ServiceNow with FullAccess rights to the SharedMailbox. This particular example uses a nested workflow call in order to simplify the workflow. |
UpdateTableRecord | Update and close the Request Item (RITM) in ServiceNow |
UpdateTableRecord | Update and close the Request in ServiceNow |
The result should be as follows:

Update Table Record explanation
For communication back to ServiceNow, the Update Table Record action in CoreView makes an API call to access specific tables in ServiceNow. It then proceeds to update or modify the records that correspond to the sys_id of the respective table record.
The business rule code in ServiceNow includes the sys_id of the Request Item and the Request as part of the workflow, along with other necessary parameters. This enables us to target the specific record that needs to be updated.
It is important to note that the custom property of the Update Table Record action utilizes the property name, which typically follows a lowercase convention in ServiceNow. This is distinct from the label or display name, which may include capitalization and spaces.
Update table record: sc_req_item
- Table Name: sc_req_item
- Sys_id: INPUT|ServiceNowID
- Custom Property 1
- Key: state
- Value: 3
- Custom Property 2
- Key: close_code
- Value: Solved (CoreView) *this is custom*
- Custom Property 3
- Key: close_notes
- Value: Shared Mailbox (INPUT|SharedMailboxName) Created via CoreView

Update table record: sc_request
- Table Name: sc_request
- Sys_id: INPUT|ServiceNowRequestID
- Custom Property 1
- Key: request_state
- Value: closed_complete
- Custom Property 2
- Key: close_notes
- Value: Shared Mailbox (INPUT|SharedMailboxName) Created by CoreView

Part 2: integrate ServiceNow
Step 1: insert the script include
The script include serves as the foundation for all workflow executions from ServiceNow to CoreView. Its purpose is to save coding effort by avoiding the need to repeat and troubleshoot the API call in multiple request forms.

This script include consists of two functions:
- one for general calls
- and another specifically designed for business rules
Show the code block for script include
//NOTE: the following details need amending in the script: authUrl, authToken, companyID, workflowUrl
var CoreViewWorkflowCall = Class.create();
CoreViewWorkflowCall.prototype = Object.extendsObject(AbstractAjaxProcessor, {
CVWorkflowCall :function(){
var CVWorkflowID = this.getParameter('sysparm_CVWorkflowID');
var CVWorkflowParams = this.getParameter('sysparm_CVWorkflowParams');
//Building the CoreView workflow call
var authUrl = 'https://www.loginportal.online/api/auth'; //url to auth to CoreView
var authToken = '**********m1K-PAFbI4lAaO'; //CoreView api auth token
var companyId = '**********bdfe1a974a345ea3042d4b'; //internal CoreView ID for company tennat
//Get an access token from CoreView
var jwtRequest = new sn_ws.RESTMessageV2();
jwtRequest.setEndpoint(authUrl);
jwtRequest.setHttpMethod('post');
jwtRequest.setRequestHeader("Accept","Application/json");
jwtRequest.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
jwtRequest.setRequestBody('{}');
jwtRequest.setRequestHeader("Authorization", "Bearer " + authToken);
var jwtResponse = jwtRequest.executeAsync();
jwtResponse.waitForResponse(60);
var jwtBody = jwtResponse.getBody();
var jwtToken = JSON.parse(jwtBody);
var jwt = jwtToken.bearerToken;
// Workflow call Variables
var workflowUrl = 'https://coreflowusapi.coreview.com';
var workflowPath = '/api/executions/';
var workflowId = CVWorkflowID; //workflow being called in CoreView passed from glideajax in client script
//Execute the call back to the CoreView api to run the workflow
var request = new sn_ws.RESTMessageV2();
request.setEndpoint(workflowUrl + workflowPath + workflowId);
request.setHttpMethod("post");
request.setRequestHeader("Accept", "Application/json");
request.setRequestHeader("Authorization", "Bearer " + jwt);
request.setRequestHeader("x-scompany", companyId);
request.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
request.setRequestBody(CVWorkflowParams); //workflow params passed from glideajax in client script, JSON was stringified before the call
var response = request.executeAsync();
response.waitForResponse(60);
var responseBody = response.getBody();
},
CVWorkflowCall_BR :function(CVWorkflowID, CVWorkflowParams){
//Building the CoreView workflow call
var authUrl = 'https://www.loginportal.online/api/auth'; //url to auth to CoreView
var authToken = '**********m1K-PAFbI4lAaO'; //CoreView api auth token
var companyId = '**********bdfe1a974a345ea3042d4b'; //internal CoreView ID for company tennat
//Get an access token from CoreView
var jwtRequest = new sn_ws.RESTMessageV2();
jwtRequest.setEndpoint(authUrl);
jwtRequest.setHttpMethod('post');
jwtRequest.setRequestHeader("Accept","Application/json");
jwtRequest.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
jwtRequest.setRequestBody('{}');
jwtRequest.setRequestHeader("Authorization", "Bearer " + authToken);
var jwtResponse = jwtRequest.executeAsync();
jwtResponse.waitForResponse(60);
var jwtBody = jwtResponse.getBody();
var jwtToken = JSON.parse(jwtBody);
var jwt = jwtToken.bearerToken;
// Workflow call Variables
var workflowUrl = 'https://coreflowusapi.coreview.com';
var workflowPath = '/api/executions/';
var workflowId = CVWorkflowID; //workflow being called in CoreView passed from glideajax in client script
//Execute the call back to the CoreView api to run the workflow
var request = new sn_ws.RESTMessageV2();
request.setEndpoint(workflowUrl + workflowPath + workflowId);
request.setHttpMethod("post");
request.setRequestHeader("Accept", "Application/json");
request.setRequestHeader("Authorization", "Bearer " + jwt);
request.setRequestHeader("x-scompany", companyId);
request.setRequestHeader("Content-type", "Application/json"); //needed for CoreView workflow v2
request.setRequestBody(CVWorkflowParams); //workflow params passed from glideajax in client script, JSON was stringified before the call
var response = request.executeAsync();
response.waitForResponse(60);
var responseBody = response.getBody();
},
type: 'CoreViewWorkflowCall'
});
Step 2: set the catalog item request form
This simple request form uses one text box for the Shared Mailbox name and a multi-row variable set for the shared mailbox membership.

Once and end-user has filled out the form and hits “Order Now/Submit”, the business rule does the processing once the item has been created in the [sc_req_item] table.
Step 3: set the business rule
The business rule processes the request once the record has been created in the [sc_req_item] table.
The code for the business rule gathers the variables from the request and sends it off to CoreView for processing. The code for this rule is very specific to this request item example and as such your code will be different for every other request. The structure will be the same, i.e. gather the values from the fields, convert it to JSON format, and then call the Script Include function to execute the API call.
Show the business rule settings
- Name: Request M365 Shared Mailbox
- Table: Request Item [sc_req_item]
- When to Run
- When: after
- Insert: checked
- Filter Conditions
- Item is ‘Request M365 Shared Mailbox’ *this is the item name of the Catalog Item that was created*
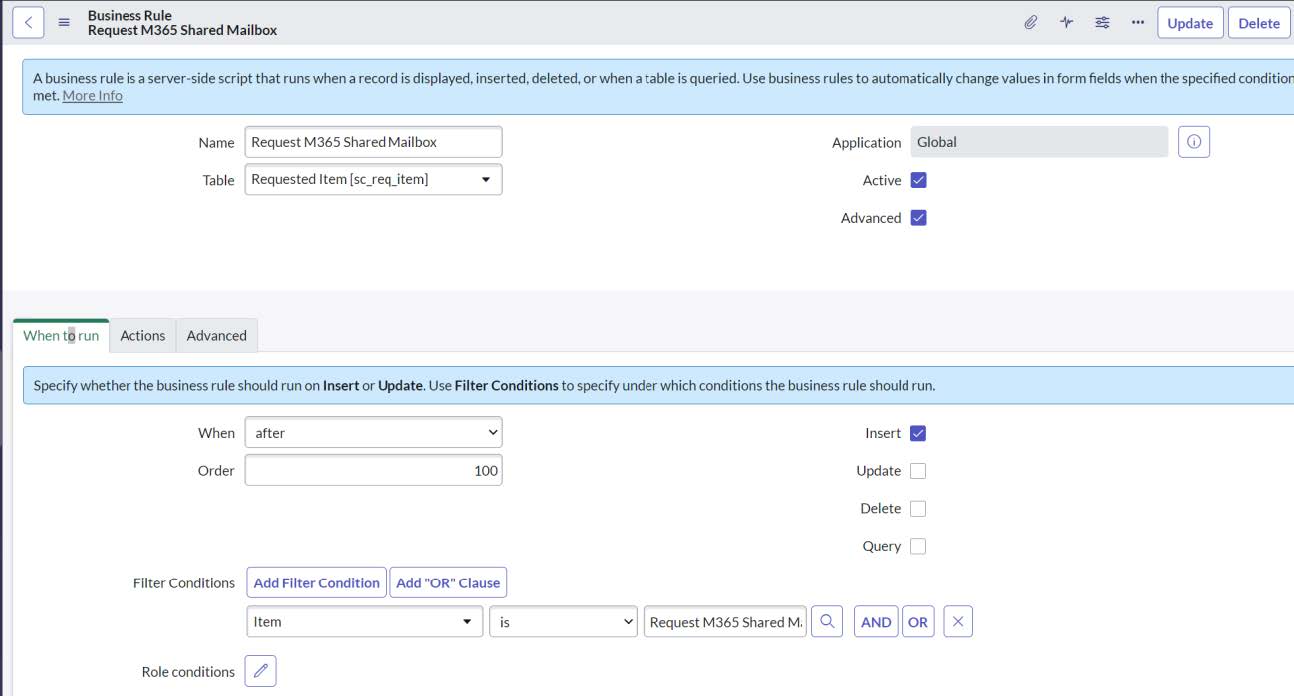
Show the code block for business rule
(function executeRule(current, previous /*null when async*/) {
// Add your code here
var ritmGR = new GlideRecord('u_m365users'); //creating pointer to user table to get user properties
var rowCount = current.variables.distributiongroupmembers.getRowCount();
var groupMembers = []; //storing the upn here
//build the array of upns for workflow input variables
for(var i = 0; i < rowCount; i++) {
ritmGR.get(current.variables.distributiongroupmembers[i].GroupMember); //goes to the record in the user table according to the sys_id of the GroupMember
groupMembers[i] = ritmGR.getValue('u_userprinciplename'); //add upn to array for use later
}
var workflowParams = {
SharedMailboxName: current.variables.DistributionGroupName.getValue(),
GroupMembers: groupMembers,
ServiceNowID: current.getUniqueValue(), //gets the sys_id of the catalog item
ServiceNowRequestID: current.request.getValue() //get the sys_id of the parent request
};
var WorkflowID = "******725-a784-23d0c1ab8c50"; //ID of workflow to execute in CoreView
var wfcall = new CoreViewWorkflowCall();
wfcall.CVWorkflowCall_BR(WorkflowID, JSON.stringify(workflowParams));
})(current, previous);